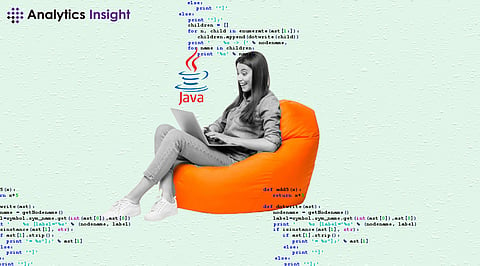
The performance should be fine-tuned for the Java applications so that the developed software does not become slow. How your application is going to be perceived by the user depends on the kind of performance tuning techniques at play. This paper discusses the best practices on Java performance tuning and gives you actionable strategies to optimize your code and system.
Before heading to tune your Java application, you have to find the bottlenecks of performance. You can utilize VisualVM, JProfiler, YourKit, or other profiling tools for doing some analysis over the application to know where exactly it spends time and what type of methods/operations area eating up its resources.
The most important concern in Java performance tuning is memory management. Here are some important techniques to enable optimum usage of memory:
Avoid Memory Leakage: Ensure all created objects are garbage collected by avoiding memory leakage. These leaks can be detected and fixed using tools such as Eclipse Memory Analyzer.
Efficient Data Structures: The usage of the right data structure within an application reduces the likelihood of constructing performance bottlenecks.
For example, use ArrayList instead of LinkedList in cases where the number of reads will be higher than the number of writes. On similar lines, use HashMap instead of Hashtable when thread safety is expected with low contention.
Fewer Objects: Avoid too many objects, more specifically within loops or typically called methods and activities. A share of objects as much as possible. For objects that are costly to create, have object pools.
Efficient CPU usage is the key to high performance. Here are a few tips to follow:
Avoid Needless Synchronization: Synchronization may introduce contention which eventually slows down the performance of your program. Always synchronize only the critical sections of your code. Modern concurrency utilities like the `java.util.concurrent` package can yield you greater performance.
Optimize Loops and Recursions: Prioritize optimizations at loops and recursive methods since they alone consume a significant amount of processing time. Apply enhanced for-loops and keep off evaluations that don't have to be inside loops.
Leverage Multi-threading: To achieve concurrency in the execution of tasks, use multithreading. But remember, too many threads always come at the cost of performance, and thread creation and management is an additional problem. So, use the thread pools through `ExecutorService` framework to efficiently handle threads.
The Input/Output operations can result in the big performance bottleneck. Here is the way to optimize them:
Use Buffered I/O: The buffered I/O streams like `BufferedReader` or `BufferedWriter` are much faster as compared to nonbuffered streams. Do buffering for file and network I/O to reduce count of I/O Operations.
Asynchronous I/O: Try to use NIO for network operations. How does it relate? It will keep all the I/O operations kicker with no blockage in the main thread.
Efficient Database Access: Make the database queries and connections more efficient. Leverage connection pooling, prepared statements, and batch processing .
Just-In-Time compiler and JVM options in Java form a substantial part of performance tuning:
Enable JIT Compiler: Ensure that the JIT compiler is turned on for optimizing byte code at runtime. By default, the HotSpot JVM does this already.
Tune JVM Options: You can further tune the performance using JVM options. You can, for example, set properties like setting heap size with –Xmx and –Xms, garbage collection with –XX:+UseG1GC, and profiling with –Xprof for monitoring performance.
Regular profiling and benchmarking are necessary to ensure peak performance. Measure with benchmarking utilities like JMH the Java Microbenchmark Harness the important sections of code. Profile regularly in your development cycle, which helps in the early detection of performance problems.
Following the best practices of coding can often avoid performance issues:
Write Clean and Efficient Code: Be readable, maintainable, and efficient. There is no need to add another level of complexity. Standard coding conventions should be followed.
Avoid Premature Optimization: First, write a correct and clean code. Optimize only where performance bottlenecks have been shown through profiling.
Document and Review Code: Document your code and perform peer code review. It will help in the possible discovery of performance issues and ensure that best practices are followed.