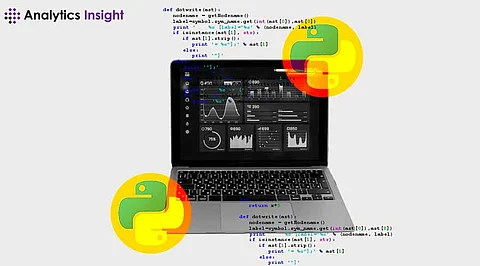
Python's popularity stems from its simplicity, versatility, and the vast ecosystem of external libraries that extend its capabilities. These libraries allow developers to perform complex tasks without writing code from scratch, making Python a powerful tool across various domains. This article delves into what external libraries are, how to install and use them, and best practices for their effective use in Python projects.
External libraries in Python are collections of pre-written code designed to extend the language's functionality. These libraries extend the functionality of Python and are not part of Python's standard library. They can be easily installed and used in your projects. They fulfill almost any need that a developer might have, right from web development and data analysis to machine learning. This saves the developer a lot of time and effort because they will not have to reinvent the wheel but instead focus on higher-level problem-solving.
For instance, if you're working on a data analysis project, you might use external libraries like pandas for data manipulation or matplotlib for data visualization. These libraries provide pre-built functions and classes that simplify complex tasks, making your development process more efficient.
The most common way to install external libraries in Python is through pip, the Python package manager. Pip allows you to easily download and install libraries from the Python Package Index (PyPI), a repository of Python packages.
1. Ensure Pip is Installed
Most installations of Python will have pip pre-installed. You can check if pip is installed using the following command in your terminal or command prompt:
pip --version
If pip is installed, this command will return the version of pip. If it's not installed, you can download and install pip from the official Python website.
2. Install a Library
To install a library, use the pip install command followed by the library name. For example, to install the requests library, which is used for making HTTP requests, you would run:
pip install requests
3. Verify Installation
After installation, you can verify that the library is installed by importing it in a Python script or interactive session:
import requests
print(requests.__version__)
This command will print the installed version of the requests library, confirming that it was successfully installed.
4. Upgrade a Library
To upgrade an existing library to the latest version, use the --upgrade flag:
pip install --upgrade requests
This command will update the requests library to its latest version.
5. Uninstall a Library
If you need to remove a library, use the pip uninstall command:
pip uninstall requests
This command will uninstall the requests library from your environment.
One of the best practices to use when working with external libraries is a virtual environment. The virtual environment is a Python isolation whereby dependencies for different projects can be managed independently. This will be ensured with the avoidance of conflicts between libraries and make sure that every means has the dependencies exactly required by it.
1. Create a Virtual Environment
You can create a virtual environment using the venv module:
python -m venv myenv
This command creates a virtual environment called myenv.
2. Activate the Virtual Environment
To activate the virtual environment:
a. In Windows, it is done through:
myenv\Scripts\activate
b. On macOS and Linux:
source myenv/bin/activate
Once activated, the virtual environment will be indicated by the environment name in your terminal prompt.
3. Install Libraries in the Virtual Environment
With the virtual environment activated, any libraries you install using pip will be confined to this environment:
pip install requests
4. Deactivate the Virtual Environment
Turn off the virtual environment:
deactivate
To make the most of external libraries in Python, consider the following best practices:
1. Research Thoroughly
The first thing you want to do before using any external library is research in their documentation, community support, and compatibility with your project's needs. Popular libraries come with extensive documentation and active communities which is very handy when trying to solve a problem.
2. Use Virtual Environments
As already said, virtual environments help with dependency management, not having different projects conflict with each other. They also make the reproducing of environments across systems much easier, ensuring that your project behaves well in the various different environments.
3. Keep Dependencies Updated
Keeping your libraries up to date makes sure your application always has the latest features, performance improvements, and security fixes. However, be cautious of breaking changes in new versions, which might affect your project. It's advisable to test your project after updating any dependencies.
4. Pin Dependencies
A requirements.txt file is best used to pin to the versions of the libraries your project depends on. That makes your project stable and reproducible across different environments. You can generate this file using:
pip freeze > requirements.txt
This command creates a requirements.txt file with the exact versions of all installed libraries, which can then be used to recreate the environment.
5. Follow Semantic Versioning
When you announce a library version, semantic versioning dictates the answers: Semantic versioning gives you an idea what kind of effects updating produces. Aids in maintaining compatibility by giving in what versions should work together. It is of normal versioning: MAJOR.MINOR.PATCH in that, respectively:
a. MAJOR: Incompatible changes.
b. MINOR: Backwards compatible functionality added.
c. PATCH: Backward compatible bug fixes.
6. Read the Documentation
Read the documentation for any library you use thoroughly. Knowing the API, features, and limitations of the library can save you time and prevent potential issues during development.
7. Test Thoroughly
When adding a new library, you should write tests to verify it works in your project as expected. It will help get the problems early and thus, ensure that the library integrates well with your codebase.
8. Contribute Back
If you find any bugs or want a new feature in an external library, please consider contributing back to the project. Open-source libraries rely on community contributions, and your input can be useful in making the potential changes for the greater good of the library.
Here are some popular external libraries across various domains:
Web Development:
a. Django: A high-level web framework that encourages rapid development and clean, pragmatic design.
b. Flask: A lightweight and very simple micro web framework.
Data Analysis and Manipulation:
a. pandas: High-performance, easy-to-use data structures and data analysis tools.
b. numpy: Scientific computing fundamental package for Python.
Machine Learning and AI:
a. scikit-learn: Machine learning library for Python with a set of various algorithms and tools.
b. TensorFlow: Google's framework for machine learning and AI, open source.
c. PyTorch: Open-source deep learning platform by Facebook.
Data Visualization:
a. matplotlib: General library for creating static, animated, and interactive visualizations.
b. seaborn: Based on matplotlib, it provides a high-level interface for drawing attractive and informative statistical graphics.
Web Scraping:
a. BeautifulSoup: Library for pulling data out of HTML and XML documents.
b. Scrapy: An open-source and collaborative web crawling framework.
Networking and APIs:
a. requests: Library for making HTTP requests simple
b. socket: Low-level networking interface.
Databases:
a. SQLAlchemy: SQL toolkit and Object-Relational Mapping (ORM) library
b. sqlite3: Python interface for SQLite Databases.
Testing:
a. pytest: A robust framework for writing and executing tests.
b. unittest: (Part of the standard library) Unit testing framework.
GUI Development:
a. tkinter: Standard GUI library for Python.
b. PyQt: Set of Python bindings for the Qt application framework.
Using external libraries in Python could actually help in hugely enhancing the coding journey by offering advanced features and capabilities and reducing workload and complexity. Virtual environments, recommended practices for updating needed dependencies, and an in-depth exploration of a library ensure that your projects will stay reliable, effective, and manageable with ease. Welcome the extensive collection of Python libraries to discover new opportunities and optimize your coding process.
1. What are external libraries in Python, and why should I use them?
External libraries in Python are collections of pre-written code that extend Python’s capabilities. They offer specialized functions, classes, or methods not available in the standard library, enabling developers to perform tasks like data analysis, machine learning, or web development efficiently. Using these libraries saves time and effort, allowing you to focus on higher-level problem-solving instead of writing code from scratch.
2. How do I install external libraries in Python?
You can install external libraries in Python using the package manager pip. First, ensure pip is installed by running pip --version in your terminal. To install a library, use the command pip install library_name, replacing "library_name" with the desired library. For example, pip install requests installs the requests library. You can also upgrade libraries with pip install --upgrade library_name and uninstall them using pip uninstall library_name.
3. What are virtual environments, and why should I use them?
Virtual environments in Python are isolated environments that allow you to manage dependencies separately for different projects. They prevent conflicts between libraries and ensure each project has its specific dependencies. By using virtual environments, you can maintain a clean and organized development environment, making it easier to manage multiple projects and avoid version conflicts between libraries.
4. How do I keep my external libraries up to date?
To keep your external libraries up to date, regularly use the command pip install --upgrade library_name for each library in your project. You can also check for updates across all installed libraries using pip list --outdated. Updating libraries ensures you benefit from the latest features, performance improvements, and security patches, but always review the changelog to avoid potential breaking changes that could affect your project.
5. What are the best practices for using external libraries in Python?
Best practices for using external libraries in Python include thoroughly researching libraries before use, utilizing virtual environments to manage dependencies, keeping libraries up to date, and pinning specific versions in a requirements.txt file. Additionally, it’s crucial to read documentation, write tests for new libraries, and contribute back to the open-source community if possible. Following these practices ensures a stable, efficient, and maintainable codebase.