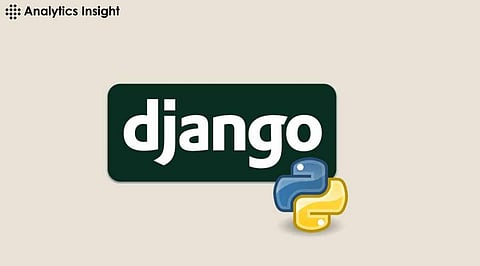
Python has emerged as one of the most popular programming languages due to its simplicity, versatility, and robust ecosystem. When it comes to web development, Django stands out as a powerful, high-level Python framework that allows developers to build web applications quickly and efficiently. This tutorial explores the essential concepts of web development using Django, providing insights into how Django works and how it can be utilized for creating dynamic websites.
Django is an open-source web framework written in Python. It was designed to help developers create web applications cleanly and pragmatically. Django follows the Model-View-Template (MVT) architectural pattern, which separates the application's data model, business logic, and user interface. Django aims to automate as much as possible to avoid redundant tasks, enabling rapid development and clean, maintainable code.
Rapid Development: Django allows developers to build and deploy web applications faster by providing reusable components and built-in tools.
Security: Django comes with built-in protection against common web vulnerabilities like cross-site scripting (XSS), SQL injection, and cross-site request forgery (CSRF).
Scalability: Django is designed to handle high traffic and large-scale applications efficiently.
Admin Interface: Django automatically generates an admin interface based on the models, allowing developers to manage data easily.
The Model-View-Template (MVT) architecture is central to Django’s design. This pattern is similar to the Model-View-Controller (MVC) used in other frameworks but with a slight difference in terminology.
The model is the part of the application that handles the database. It defines the data structure and provides methods to interact with the database. In Django, models are written as Python classes and mapped to database tables.
The view processes user requests and returns the appropriate response. In Django, views are Python functions that handle the logic of the application. Views retrieve data from models and pass it to the templates.
The template represents the user interface of the web application. Django uses templates to define how the data is presented to the user. Templates are typically HTML files that include dynamic content using Django’s template language.
This separation of concerns allows for cleaner, more maintainable code.
Setting up Django requires installing Python and the Django framework. Once the installation is complete, a new Django project can be created. A Django project is a collection of settings for an instance of Django, including configuration for the database, Django apps, and static files.
The basic steps to set up Django include:
The first step involves installing the Django framework. Django can be installed using Python’s package manager.
A new Django project can be created. This command creates a directory structure with necessary configuration files and directories.
After creating the project, the Django development server can be run. This server is used to test the application locally.
Once the project is set up, Django will have created several files, including a settings.py file, which contains all the project settings, and a urls.py file, which maps URLs to views.
Django projects are made up of multiple apps. Each app in Django is a self-contained module with its models, views, templates, and static files. This modular design helps in organizing code, making it easier to manage large projects.
The process of creating an app involves the following:
After setting up the project, a new app can be created within the project. Each app has a specific functionality or feature within the project.
Models define the structure of the database and are written as Python classes. Django’s ORM (Object-Relational Mapping) system automatically handles the mapping between these Python classes and database tables.
Once the models are defined, Django provides a migration system to create and modify the database schema. Migrations allow for changes to be made to the database without losing data.
Django automatically generates an admin interface based on the models. The admin interface is a powerful tool for managing application data.
In Django, URL routing is handled by defining URL patterns in the urls.py file. The URL patterns map specific URLs to views, which are Python functions that handle the logic of the application.
Each URL in the application is associated with a specific view. Django uses regular expressions to match URLs to their corresponding views. This allows for flexible routing and dynamic URLs.
Views handle the business logic of the application. They receive HTTP requests and return HTTP responses. Views can render templates, retrieve data from the database, and process user input.
Django’s template system allows for the separation of HTML from Python code. Templates are HTML files that include placeholders for dynamic content. Django templates use a special syntax to include variables, loops, and conditional statements.
Django’s template language provides various features for rendering dynamic content, such as including variables and performing logic directly in the HTML.
Template inheritance allows for the reuse of common layout elements. A base template can be defined, and other templates can extend it. This keeps the code DRY (Don’t Repeat Yourself) and promotes maintainability.
Handling user input is essential in web applications, and Django makes it easy with its form system. Django’s form-handling framework allows developers to define forms, validate input, and process the data.
Forms in Django can be created either manually or using Django’s built-in form classes. Forms are used to collect user input and submit it to the server.
Django automatically validates form input, ensuring that only valid data is processed. Custom validation rules can also be implemented.
Once a form is submitted, Django views processes the input and saves it to the database or performs other actions. Django provides built-in methods for handling form submissions securely and efficiently.
Django’s ORM allows developers to interact with the database using Python code instead of writing raw SQL queries. This makes database management simpler and more intuitive.
Each model in Django corresponds to a table in the database. Django’s ORM provides methods for creating, retrieving, updating, and deleting data.
Django ORM allows for complex queries using Python syntax. These queries retrieve data from the database and can filter, order, and group results.
When models are updated, Django’s migration system ensures that the database schema is updated without affecting the existing data.
Django provides a straightforward way to manage static files (like CSS, JavaScript, and images) and user-uploaded media files.
Static files, such as CSS and JavaScript, are stored separately from the templates. Django manages the collection and serving of static files during development and production.
User-uploaded files, such as images and documents, are managed using Django’s media handling system. Media files are stored in a separate directory and linked to the corresponding models.
Django offers a powerful and efficient framework for web development, allowing developers to create complex web applications quickly. Its built-in tools, modular structure, and ORM system simplify many aspects of development, making it one of the most popular frameworks for Python web development. With Django, developers can focus on writing clean, maintainable code while leveraging the framework’s robust features to build scalable and secure web applications.