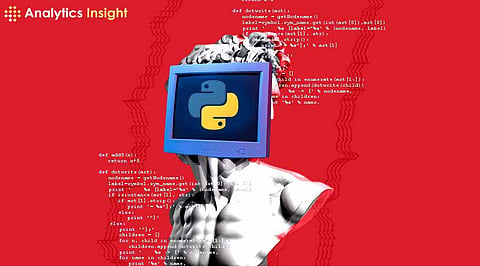
REST (Representational State Transfer) is an architectural style for designing networked applications. A REST API allows different systems to communicate over HTTP by defining a set of rules and conventions. RESTful APIs use standard HTTP methods such as GET, POST, PUT, and DELETE to perform operations on resources, which are typically represented in JSON format. These APIs are stateless, meaning each request from a client to a server must contain all the information needed to understand and process the request.
However, in order to begin constructing a REST API with Flask, there are requirements of the development environment that can be prepared beforehand. Follow these steps to get started:
You need to have Python installed in your system. You can get Python from the official Python website. Once you've installed it, just check if your installation is successful by running this on your terminal or Command Prompt:
bash
Copy code
python --version
It is a good practice to start off by creating a virtual environment so that you can handle project dependencies and refrain from conflicts with other projects. This will be done by running the following commands:
bash
Copy code
python -m venv venv
source venv/bin/activate # On Windows, use `venv\Scripts\activate`
Flask is minimal and lightweight; it's a micro-framework for Python based on Werkzeug and Jinja 2. Installation of Flask can be done with a package available in Python, which is a package manager; that is, pip. It's as simple as the command shown below:
bash
Copy code
pip install Flask
Flask-RESTful is an extension to Flask that makes the development of simple REST APIs much easier. The extension provides a structured way for defining resources and how they should be dealt with when it comes to HTTP methods. First, you need to install it with pip:
bash
Copy code
pip install flask-restful
Setting up the environment in place, let us start by creating a very basic REST API with Flask. We are going to create an API that shall be used to manage a list of books. It is proposed that each book will be characterized by an ID, title and the author.
Let's create a new file, app.py, and add the following code inside it to set up the Flask application:
python
Copy code
from flask import Flask, request, jsonify
from flask_restful import Resource, Api
app = Flask(__name__)
api = Api(app)
books = []
class Book(Resource):
def get(self):
return jsonify(books)
def post(self):
new_book = request.get_json()
books.append(new_book)
return jsonify(new_book), 201
api.add_resource(Book, '/books')
if __name__ == '__main__':
app.run(debug=True)
In this code:
a. Using the necessary packages. We are interested in Flask, request and jsonify from Flask, and Resource and Api from Flask-RESTful.
b. We define resource class Book with two methods: Get and post is such an approach. The get method returns the list of books. The post method is to add a new book in the list of the books.
c. We create the new resource named Books, and its URL will be api/[application name]/books.
d. Finally, we start the application in debug mode.
Now that we have the basic Flask application, run it with the following command in your terminal:
bash
Copy code
python app.py
Now let us extend more operations to our API for the operations of reading, modifying and erasing single entities, here books.
You can use tools like Postman or curl to test your API. To illustrate how to add a new book, you can send a POST request with the following JSON payload:
json
Copy code
{
"id": 1,
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald"
}
To retrieve the list of books, send a GET request to the same URL.
Let us add more functionality to our API, this time for retrieving, updating and deleting individual books.
Until now, GET was the only HTTP method available in our Book resource. Let's add support for other HTTP methods:
python
Copy code
class Book(Resource):
def get(self, book_id=None):
if book_id is None:
return jsonify(books)
for book in books:
if book['id'] == book_id:
return jsonify(book)
return {'message': 'Book not found'}, 404
def post(self):
new_book = request.get_json()
books.append(new_book)
return jsonify(new_book), 201
def put(self, book_id):
updated_book = request.get_json()
for book in books:
if book['id'] == book_id:
book.update(updated_book)
return jsonify(book)
return {'message': 'Book not found'}, 404
def delete(self, book_id):
global books
books = [book for book in books if book['id'] != book_id]
return {'message': 'Book deleted'}
api.add_resource(Book, '/books', '/books/<int:book_id>')
In this code:
a. The class get method has also been enhanced to return an individual book if a book_id is placed into the method otherwise, the list of all books is returned.
b. Added a new Document Method to retain the overall figure of the books in the new Document object in the statement if the book_id matches.
c. Create the delete method that is to be used in deleting a book from the list by book_id.
With such methods above included, our API should now be in a better position to do more meaningful operations on the book resources.
Using Postman or `curl` try to perform the actions that are to be observed under the new functionality. For example, to update a book, send a PUT request to http: whose JSON payload has been refreshed to account for shaking. However, as it is now, it is 127. 0. 0. 1:5000/books/1. In case a book has been deleted, one would only have to make a DELETE request to the same URL.
Making REST APIs using Flask is an easy and strong affair due to its friendly nature and flexibility. If you follow most of the best practices explained in this article, you might end up with a good enough REST API that can handle data and do much of what you expect of it. Flask-RESTful makes the process even smoother by offering a systematic approach to defining data and managing HTTP requests.
Armed by the information you have received from this tutorial, you are now prepared to dive into more sophisticated REST APIs that access databases, perform authentication of users, and integrate other systems. Flask's versatility enables you to effortlessly incorporate new features as your project expands, making it a great option for everything from small-scale projects to large-scale web applications.
Even if you are getting started and have quite a bit of development under your belt, getting really good at building REST APIs with Flask opens doors to a lot more if you really want to get into things and start exploring a good number of functionalities. Build on top of this base, and it results in the creation of robust web services that have the potential to scale and evolve responsive to needs.
1. What is Flask, and why is it used for building REST APIs?
Flask is a lightweight Python micro-framework that simplifies the process of creating web applications and RESTful APIs. It’s favored for its flexibility, minimalistic approach, and ease of use. Flask allows developers to build scalable web services quickly, using Python’s powerful capabilities. With extensions like Flask-RESTful, it provides a structured way to define resources and handle HTTP methods, making it ideal for building REST APIs efficiently.
2. What is a REST API, and how does it work?
A REST (Representational State Transfer) API is an architectural style for designing networked applications. It enables different systems to communicate over HTTP by following a set of conventions. RESTful APIs use standard HTTP methods like GET, POST, PUT, and DELETE to perform operations on resources, typically represented in JSON format. They are stateless, meaning each request contains all the information needed to understand and process the request independently.
3. How do I set up a Python environment to build REST APIs with Flask?
To set up a Python environment for building REST APIs with Flask, first, install Python from the official website. Next, create a virtual environment using python -m venv venv and activate it. Install Flask using pip install Flask, followed by installing Flask-RESTful with pip install flask-restful. This setup isolates your project dependencies, ensuring a clean and manageable environment for developing your Flask-based REST API.
4. How do I create a simple REST API with Flask?
To create a simple REST API with Flask, start by importing Flask and Flask-RESTful. Define a resource class with methods like GET and POST to manage data, such as a list of books. Add this resource to your API with an endpoint, then run the Flask application. Clients can interact with the API by sending HTTP requests to perform operations like retrieving or adding data. Flask handles routing and request processing.
5. What tools can I use to test my Flask REST API?
To test your Flask REST API, you can use tools like Postman, a popular GUI tool that allows you to send HTTP requests and view responses easily. Alternatively, you can use curl, a command-line tool for sending HTTP requests from your terminal. These tools enable you to simulate client interactions with your API, such as sending GET, POST, PUT, or DELETE requests, and verifying the correct functioning of your endpoints.
6. How can I add more functionality to my Flask REST API?
You can add more functionality to your Flask REST API by extending resource classes to handle additional HTTP methods like PUT and DELETE. This allows you to update and delete specific resources. You can also integrate database support using libraries like SQLAlchemy, implement authentication, or use Flask extensions to manage sessions, handle errors, or log activities. Flask’s modular design lets you build complex APIs while maintaining code simplicity.
7. What are Flask-RESTful and its benefits in API development?
Flask-RESTful is an extension for Flask that simplifies building REST APIs by providing tools for creating resource-oriented APIs quickly. It offers a structured approach to defining resources, handling HTTP methods, and organizing routes. With Flask-RESTful, you can manage complex APIs more efficiently, thanks to its features like request parsing, automatic JSON responses, and easy integration with Flask’s existing components. It’s ideal for developers looking for simplicity and scalability in API development.
8. How can I deploy my Flask REST API to production?
To deploy your Flask REST API to production, you can use platforms like Heroku, AWS, or Google Cloud. First, ensure your application is production-ready by configuring environment variables, securing API endpoints, and optimizing performance. Package your app with a requirements.txt file and use a WSGI server like Gunicorn for handling production traffic. Push your code to the hosting platform, configure the deployment settings, and your API will be accessible online.