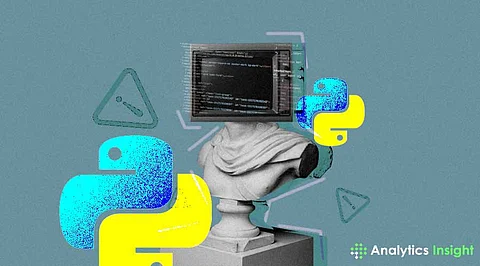
Python is widely known for its simplicity and readability. With each new version, Python continues to introduce features that enhance these qualities, making code cleaner and more efficient. One such feature is the "f-string" or formatted string literals, introduced in Python 3.6. F-strings have quickly become the preferred way to format strings due to their simplicity and performance advantages over older methods like % formatting and str.format().
F-strings, short for formatted string literals, allow variables and expressions to be embedded directly into a string by placing them inside curly braces {}. These strings are prefixed with an f or F, which signals Python to interpret the string as an f-string. This approach provides a more readable, concise, and efficient way to format strings compared to other methods.
Using f-strings comes with multiple benefits, especially when writing and maintaining Python code.
F-strings eliminate the need for cumbersome concatenations and the .format() method, making code easier to read. With f-strings, each variable or expression appears within the text where it belongs, allowing readers to understand the intent immediately.
The syntax of f-strings is shorter and more direct. F-strings embed variables directly, reducing the number of parentheses, commas, and other symbols needed in the code. For larger projects, this conciseness can significantly simplify lines of code.
F-strings outperform both % formatting and str.format(), especially with multiple variables. Performance data from recent tests show that f-strings execute about 30% faster than .format(). This improvement is particularly beneficial when working with larger datasets or loops that require frequent string formatting.
F-strings support embedding not only variables but also complex expressions. Mathematical operations, function calls, and conditionals can all be included within f-strings. This flexibility allows for more dynamic and efficient string creation, useful in many real-world applications.
F-strings are versatile and can be used in numerous scenarios in Python programming.
In many cases, f-strings are used to insert variable values directly into a string. This use is common when creating dynamic messages or logging outputs, as f-strings allow easy insertion of values without additional code.
F-strings go beyond simple variable interpolation by allowing expressions inside curly braces. This capability makes it possible to perform operations directly within the string, eliminating the need to declare intermediate variables. This is helpful for calculations, string manipulation, and conditional expressions that need to be formatted directly in the output.
Python f-strings support format specifications for numbers and dates, providing greater control over output. For instance, f-strings can format numbers with commas, decimal precision, or even currency symbols. Similarly, dates can be formatted to various standards, making f-strings a powerful tool for creating readable and user-friendly data displays.
F-strings support multi-line strings using triple quotes (""" ... """). This feature allows for easy embedding of variables and expressions across multiple lines. Multi-line f-strings are especially useful for creating structured text outputs, like HTML, JSON, or other markup languages.
Although f-strings allow complex expressions, keeping expressions simple improves readability. Using complex logic within an f-string can make the code harder to understand. Whenever possible, perform calculations outside the f-string and use the result within it.
When formatting numbers, dates, or other data, using format specifiers ensures the output is clear and consistent. Common specifiers include .2f for two decimal places and , for thousand separators. These specifiers make numerical data more readable, especially when presenting financial or statistical information.
Functions and f-strings work well together. If a section of text or calculation needs to be reused, placing it in a function keeps the code clean and organized. F-strings can then call these functions directly, reducing redundancy and improving maintainability.
F-strings are not recommended for constructing SQL queries due to security concerns like SQL injection. Instead, use parameterized queries or ORM (Object-Relational Mapping) libraries, which provide a safe way to handle user input in SQL statements. Avoiding f-strings in these cases helps protect applications from vulnerabilities.
F-strings offer notable improvements over older formatting methods, such as % formatting and str.format().
% Formatting: This was Python’s original string formatting method. While still valid, it requires more syntax and doesn’t support complex expressions. F-strings are generally faster and more readable.
str.format(): This method improved on % formatting by allowing positional arguments and more control. However, str.format() requires more syntax and is generally slower than f-strings.
In most cases, f-strings outperform both alternatives in terms of readability, speed, and versatility.
F-strings are useful for logging, where dynamic data needs to be embedded in log messages. With f-strings, logging statements become more concise, and developers can include expressions and function calls directly. This approach makes debugging and tracking program flow easier.
Reports often require formatted data presentation, such as tables, charts, or summaries. F-strings simplify the process by allowing precise control over how numbers and dates are displayed. Formatting options make f-strings ideal for creating structured, readable reports with minimal code.
Many applications involve generating structured text formats, like HTML or JSON. F-strings make it easy to dynamically insert data into these formats without complex concatenation. By using multi-line f-strings, developers can create templates for structured text that are both readable and maintainable.
When working with large datasets, efficient string formatting becomes essential. F-strings offer the performance needed to handle frequent updates and recalculations. Financial data, for example, often requires real-time formatting, which f-strings handle more efficiently than older methods.
With Python’s ongoing development, f-strings have gained new capabilities in recent versions. In Python 3.12, error handling for f-strings has improved, making it easier to spot syntax errors. This enhancement ensures developers can confidently use f-strings for complex string manipulations, knowing the debugging process is straightforward.
Python’s commitment to enhancing f-strings suggests that they will continue to be a core feature in the language. Given their speed, readability, and flexibility, f-strings are expected to remain the go-to method for string formatting in future Python versions.
F-strings are one of Python’s most powerful tools for simplifying code and improving performance. By allowing direct embedding of variables and expressions, f-strings enhance readability and reduce syntax complexity. Their flexibility in handling numbers, dates, and multi-line strings makes them suitable for a wide range of applications. As Python evolves, f-strings continue to receive updates, solidifying their place as the preferred method for string formatting in Python. Using f-strings wisely can lead to cleaner, more maintainable code that performs efficiently across various applications.