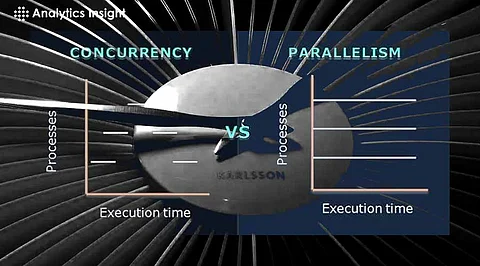
Concurrency and parallelism are two techniques for managing multiple tasks in a program, but they operate differently. Understanding the distinction between them in Python helps developers write optimized code tailored to specific tasks.
Concurrency allows multiple tasks to make progress without finishing them one by one. However, they don't necessarily execute at the same time. Concurrency achieves multitasking by rapidly switching between tasks, giving the appearance of simultaneous execution. In Python, concurrency is commonly implemented using multi-threading or asynchronous programming.
For example, when a program needs to handle tasks like reading a file, waiting for data from the internet, or writing to a database, concurrency is particularly useful. The program can continue executing other tasks while waiting for these slower I/O operations to complete. It is especially effective for I/O-bound tasks, where waiting for external events (like network responses) takes up a significant amount of time.
Concurrency doesn't require multiple processors or cores. A single-core CPU can still manage concurrent tasks by switching between them. In Python, the asyncio library is a popular choice for concurrency, particularly when dealing with non-blocking I/O.
Parallelism, on the other hand, involves executing multiple tasks at the same time. To achieve true parallelism, the system must have multiple CPU cores or processors. Each core can handle a separate task, allowing them to run simultaneously without interference.
In Python, parallelism is generally achieved using multiprocessing, where multiple processes run independently across different cores. This is highly effective for CPU-bound tasks, such as large computations or data analysis. Unlike concurrency, where tasks share a single processing unit, parallelism allows tasks to use separate cores, thus fully utilizing the system's processing power.
Parallelism is perfect for tasks that involve heavy computation and benefit from being split into smaller, independent units. Python's multiprocessing module is commonly used for parallelism, as it allows different processes to run in isolation on separate cores, bypassing the limitations of the Global Interpreter Lock (GIL).
Concurrency: The system switches between tasks, but they do not run at the same time. The illusion of multitasking is created through task interleaving.
Parallelism: Tasks truly run at the same time on different processors or cores.
Concurrency: Best for I/O-bound tasks like waiting for data from a network or reading a large file. These tasks spend a lot of time waiting for input/output, so switching between them improves efficiency.
Parallelism: Ideal for CPU-bound tasks, which are computation-heavy, like number crunching, data processing, or machine learning training. These tasks require significant processing power.
Concurrency: Uses a single CPU and shares it between tasks. CPU usage is not continuous for each task, and memory consumption remains lower.
Parallelism: Takes advantage of multiple CPU cores, resulting in better CPU utilization and faster execution of tasks. However, this also leads to higher memory usage because each task may require its own memory space.
Concurrency: Tasks may depend on each other or external factors, like a server response. The tasks overlap, but they do not need to run simultaneously.
Parallelism: Tasks are usually independent of each other. Each task runs in isolation, making it ideal for tasks that can be broken into smaller, self-contained units.
Concurrency in Python is often achieved using multi-threading, where different threads run concurrently. Each thread is an independent flow of execution within the same program. The operating system switches between threads, allowing multiple tasks to make progress simultaneously.
However, Python has a limitation known as the Global Interpreter Lock (GIL). The GIL ensures that only one thread executes Python bytecode at any given time, which prevents true parallelism in CPU-bound tasks. Although multi-threading can still be useful for I/O-bound tasks, it does not provide performance benefits for CPU-intensive operations.
Asynchronous programming is another form of concurrency, and Python's asyncio library is a popular tool for this. In asynchronous programming, the program can perform non-blocking I/O tasks without waiting for their completion. The program can execute other tasks while waiting for an I/O operation to finish.
This is particularly useful for web servers, database operations, or any task that involves waiting for an external resource. Async programming improves responsiveness and efficiency in these scenarios.
Python's multiprocessing module allows for true parallelism by creating separate processes that can run independently on different CPU cores. Unlike threads, processes do not share memory space, meaning they run in complete isolation. This ensures that multiple processes can execute simultaneously, taking full advantage of the system's multi-core architecture.
Multiprocessing is perfect for CPU-bound tasks where dividing the workload into smaller tasks allows them to run concurrently across multiple cores. This leads to faster execution and improved performance.
For tasks that require even more processing power, distributed computing is an option. Python frameworks like Dask or Ray allow for parallel execution across multiple machines. This enables large-scale parallelism, where tasks are distributed across many nodes in a cluster. Distributed computing is ideal for big data applications, machine learning, and scientific computing.
Understanding when to apply concurrency or parallelism is key to optimizing performance. The type of task—whether I/O-bound or CPU-bound—will determine the best approach.
Concurrency is ideal for I/O-bound tasks. These tasks involve waiting for external resources, and concurrency allows for efficient switching between tasks without significant computational overhead.
Parallelism is best suited for CPU-bound tasks that require intensive processing. By spreading the workload across multiple cores, parallelism speeds up execution and fully utilizes the hardware's processing capabilities.
Consider a web scraping program that downloads images from multiple websites. This program needs to both download and process the images. Concurrency is ideal for the downloading part, as it involves waiting for the images to be fetched from the web. Asynchronous programming or multi-threading can handle this efficiently.
Once the images are downloaded, they need to be processed, such as resizing or applying filters. This part of the task is CPU-bound, and parallelism using multiprocessing can speed up image processing by utilizing multiple cores.
Concurrency and parallelism are both crucial techniques for improving the performance of Python programs. While concurrency focuses on managing multiple tasks through interleaving and overlapping, parallelism is about executing tasks simultaneously on multiple cores.
Concurrency is the go-to solution for I/O-bound tasks, where efficiency is gained by switching between tasks rather than running them at the same time. Parallelism, however, shines when dealing with CPU-bound tasks, where true simultaneous execution leads to faster processing.
Knowing when to use concurrency or parallelism is key to writing efficient, scalable, and high-performing Python applications. Understanding the strengths and limitations of each approach allows developers to optimize their programs based on the specific demands of their tasks.