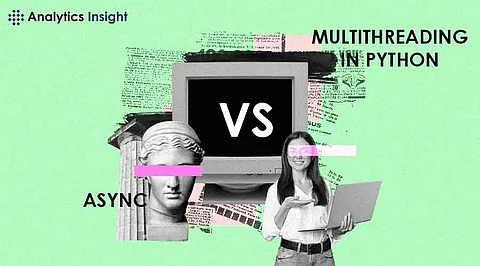
When it comes to managing concurrent tasks in Python, developers often face a choice between asynchronous programming (async) and multithreading. Both approaches have their strengths and weaknesses, and the right choice depends on the specific requirements of the task at hand. In this article, we will explore the key differences between async and multithreading, their use cases, and which one might be better suited for your project.
Understanding Asynchronous Programming (Async)
Asynchronous programming, or async, is a programming paradigm that allows you to write code that runs concurrently, without blocking the execution of other tasks. In Python, the `asyncio` library is commonly used to write asynchronous code using the `async` and `await` keywords. The primary goal of async programming is to perform non-blocking I/O operations, such as reading from a file, querying a database, or making HTTP requests, while other tasks continue to run.
Async works by using an event loop that continuously checks for tasks that are ready to be executed. When a task is waiting for an I/O operation to complete, the event loop suspends it and switches to another task. Once the I/O operation is complete, the event loop resumes the suspended task from where it left off. This approach allows for efficient use of resources and can handle a large number of I/O-bound tasks concurrently.
Non-blocking I/O: Async is highly efficient for tasks that involve a lot of waiting for external resources, such as network calls or disk I/O.
Scalability: Since async does not create multiple threads, it is lightweight and can scale well, especially for applications that need to handle thousands of connections simultaneously.
Simpler Error Handling: With async, error handling is often more straightforward compared to multithreading, as there is no need to worry about race conditions or deadlocks.
Not Suitable for CPU-bound Tasks: Async is not ideal for CPU-intensive tasks that require heavy computation, as it relies on a single-threaded event loop.
Steeper Learning Curve: Async programming can be more complex to learn and implement, especially for developers who are not familiar with the async/await syntax and event-driven programming.
Limited Libraries: Some Python libraries are not designed to work with async, which can limit your choices when building an async application.
Multithreading is a technique that allows multiple threads to run concurrently within the same process. In Python, the `threading` module provides a way to create and manage threads. Each thread runs independently, and the operating system manages the execution and scheduling of these threads.
In a multithreaded program, multiple threads share the same memory space and can run simultaneously on multiple CPU cores. The operating system schedules threads to run on different cores, allowing for true parallelism in CPU-bound tasks. Threads can communicate and share data with each other, but this also introduces the risk of race conditions and requires careful synchronization
Suitable for CPU-bound Tasks: Multithreading is ideal for CPU-intensive tasks that require parallel execution, such as image processing, data analysis, or machine learning computations.
Simpler to Implement for Beginners: For developers new to concurrent programming, multithreading can be easier to grasp, as it follows a more traditional programming model.
Wide Library Support: Most Python libraries are compatible with multithreading, making it a versatile choice for various types of applications.
Thread Safety Issues: Multithreading can introduce complex issues such as race conditions, deadlocks, and resource contention, which require careful handling using locks or semaphores.
Higher Memory Overhead: Each thread consumes additional memory, which can become a bottleneck when scaling to a large number of threads.
Global Interpreter Lock (GIL): Python’s Global Interpreter Lock (GIL) prevents multiple native threads from executing Python bytecodes at once, which can limit the performance benefits of multithreading in CPU-bound applications.
The choice between async and multithreading depends largely on the nature of the tasks your application needs to perform:
Choose Async if your application is primarily I/O-bound and needs to handle a large number of concurrent connections, such as web servers, chat applications, or real-time data feeds. Async provides an efficient way to manage multiple I/O operations without the overhead of managing multiple threads.
Choose Multithreading if your application involves CPU-bound tasks that can benefit from parallel execution, such as data processing, scientific computations, or image rendering. Multithreading allows you to leverage multiple CPU cores to perform computations faster.
In some cases, you may need to combine both approaches. For example, a web server might use async to handle incoming requests and multithreading to perform background data processing.
Both async and multithreading are powerful tools for managing concurrency in Python. Understanding the strengths and limitations of each approach will help you choose the best strategy for your specific use case. By leveraging the right concurrency model, you can build more efficient and responsive applications that meet the demands of today's dynamic computing environment.