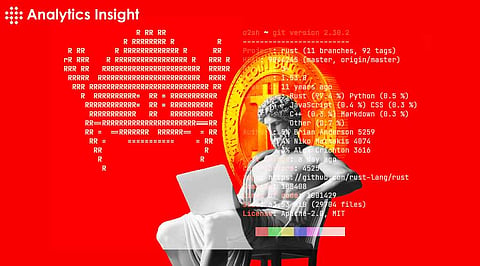
Rust is a systems programming language that promises memory safety, speed, and concurrency. It guarantees memory safety without using a garbage collector, though it's also notoriously hard to learn for the beginner. Keeping this in mind, here are ten basic tips that can help you get started with Rust programming.
One of the most distinctive features of Rust is its ownership model. Mastering this model is crucial for programming safety and idiomatic memory borrowing. Every value in Rust has an owner. If its owner goes out of scope, Rust automatically deallocates the memory and avoids memory leaks.
First, pay attention to the rules of ownership. Such rules allow you to write safe and efficient code and avoid the most common pitfalls: dangling pointers. Rust also uses borrowing: while one variable is using another, it is temporarily lending the data out, instead of giving it away. The ability to borrow data and the guarantees around that ensure that you won't clone the data unnecessarily, which often brings performance wins and fewer bugs.
Rust has an incredible built-in tool called 'cargo', designed to serve as a package manager and build system in one. It is a basic tool that you will need for your workflow in Rust; therefore, now is a good time to learn how to use it. You can create new projects with it, build, run tests, or manage dependencies.
Knowing a few standard 'cargo' commands will save you a great amount of time, keeping your projects organized. Here's how a few basic commands like 'cargo new', 'cargo run', and 'cargo test' will get the kinks out in your development.
Rust has an enormous ecosystem of libraries, called "crates," which you can use to extend your projects. Generally, as a beginning programmer, it's a bad idea to try and build anything yourself. Leverage existing crates to perform common activities like serializing data, sending HTTP requests, or even just manipulating strings. Using these crates will save you legwork and let you focus on solving the specific problems in your project.
In Rust, semantics around error handling is not an afterthought but a foreground concept. Rust does not use exceptions as many languages do. Rust handles errors implicitly and safely using types like 'Result' and 'Option'. Mastering how Rust expects you to handle errors will make your code more solid and resistant. Also, using unwrap() as a way of saving time by skipping errors entirely will end up crashing a program in production. Instead, get used to the structured way Rust handles errors from the very beginning.
Rust emphasizes immutability by default. That means, by default, variables are never changed; they become mutable only if explicitly specified. This helps to avoid errors since data will never change unexpectedly.
Or at least, it should. You'll often find yourself wanting to make variables mutable as a matter of course when first starting out. Try to resist this urge, and take Rust's defaults to heart. This leads to easier-to-understand, and therefore safer, code. It forces you to think extra hard about exactly when data is changing. Fewer unintended side effects mean less debugging.
Pattern matching enables you to concisely handle various cases in your code. Rust's 'match' expression replaces complex if-else chains and provides more granular control over how your code functions with respect to different data inputs. When working with data that might have multiple forms—like function return values or different types of enum variants, for instance—pattern matching makes your code concise, readable, and safe because Rust will check that you've covered every possible value.
Lifetimes in Rust help the compiler ensure that references to data are valid for as long as you need them, and no longer. At first, lifetimes can seem complex, but understanding them early on will help you write more efficient and safe code.
Start by learning how Rust automatically infers lifetimes, and then move on to explicitly specifying lifetimes in more complex scenarios. Lifetimes play a key role in borrowing and references, so it's worth investing the time to understand them well.
Testing is intricately woven into the development process in Rust. Rust has a built-in test framework, which motivates one to write tests during development. By writing tests well in advance and running them more frequently you can catch bugs before they grow big. Testing will help you make sure that your code works the way you want it to, and when you change something, the code stays that way. Regularly run your tests with cargo test to make sure your code behaves as you expect.
Rust has excellent documentation for both the standard library and in general for crates. While working on projects, make it a habit to look up functions, methods, and patterns in the official documentation. Documentation is very often updated in Rust and mostly clear, thus making it perfect for those starting their work with Rust.
Using Cargo doc you can create documentation for your own programs and view an HTML version of the same to make it easier to see what your code does. The documentation will support you learning how to use the standard library, and how to write idiomatic Rust code.
Conclusion: Learning Rust is not easy, but building your foundation around the core concepts of ownership, error handling, and lifetimes will get you most of the way there. Keep the tool belt filled to the brim along the way with the Cargo package manager, pattern matching, and testing, and reach out to the community for help whenever you need it. With patience and practice will come the ability to confidently write high-performance, memory-safe applications that are a delight to write.