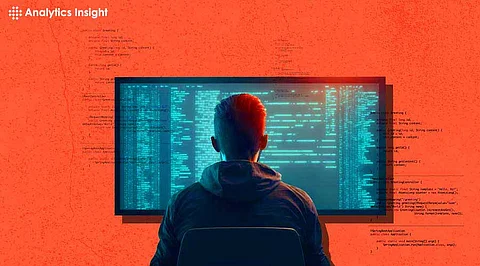
Reactive programming is a paradigm that embraces asynchronous data streams. It’s about handling data and events in a non-blocking way. This approach suits modern application requirements. With users expecting real-time updates, reactive programming enables smooth user experiences. In this article, we'll explore its core concepts and real-world use cases.
Reactive programming builds on four principles:
Asynchronous Data Streams: It treats data as continuous streams. These streams can emit values over time, making it easier to handle dynamic data.
Event-Driven Architecture: In reactive programming, changes trigger events. Every time a stream updates, it notifies subscribers. This approach reduces the complexity of managing state and synchronization.
Non-Blocking Operations: Non-blocking calls ensure threads don’t wait for a task to complete. It makes the system more responsive and scalable.
Backpressure Handling: It’s about managing the flow of data between producer and consumer. If the consumer is slower, backpressure ensures that the producer won’t overwhelm it with data.
These principles make reactive programming ideal for building applications that require high concurrency and low latency.
To better understand how it works, we need to look at its main components:
Observable (or Publisher): This is the source of data. It emits data streams that can be observed.
Observer (or Subscriber): It subscribes to an observable. Whenever the observable emits data, the observer reacts.
Operators: These are functions that can modify or transform data streams. Operators filter, map, or combine different streams.
Schedulers: These define the context in which a task runs. Schedulers control threading and concurrency.
Together, these components create a powerful toolkit for building reactive systems.
The concept of reactive programming is well-captured by libraries like Reactive Extensions (Rx). Rx has implementations for various languages such as RxJava, RxJS, and Rx.NET.
Composability: Rx allows you to compose data streams easily.
Error Handling: It has built-in error handling mechanisms, making it robust.
Backpressure Support: Rx provides tools to handle backpressure, ensuring smooth data flow.
These features make Rx a popular choice for developers building reactive systems.
Reactive programming also has a functional variant, called Functional Reactive Programming (FRP). FRP combines reactive programming principles with functional programming paradigms.
Reactive Programming: It focuses on events, data streams, and reactions.
FRP: It combines these concepts with pure functions and immutability.
FRP is more prevalent in UI frameworks like Elm and React. It allows developers to express complex UI logic in a declarative style.
Reactive programming has found its way into various domains. Below are some common use cases:
Reactive programming is perfect for handling complex UI interactions. It allows UI elements to react to data changes in real time.
For example, in a search box, every keystroke can be treated as an event. The system can react to these events by updating suggestions dynamically. This makes the application feel more responsive.
Frameworks like React and Angular embrace these reactive principles. They help build UIs that update seamlessly based on user interactions.
Reactive programming is a natural fit for real-time data processing. Systems like stock trading platforms, online games, and social media apps generate large volumes of data.
With reactive programming, these systems can process and display information in real time. Apache Kafka, combined with RxJava or Akka Streams, can build such systems. It ensures that data is handled efficiently, with no delays.
Microservices often need to communicate asynchronously. Reactive programming helps here by enabling non-blocking communication.
Libraries like Spring WebFlux and Akka offer reactive APIs for building microservices. This approach makes it easy to build scalable services. It also reduces latency when communicating between different microservices.
IoT devices generate a massive amount of data continuously. This data needs real-time processing. Reactive programming helps process and analyze IoT data streams efficiently.
For instance, a smart home system can react to data from sensors. If a motion sensor detects movement, it can trigger actions like turning on lights.
Reactive frameworks like RxJava or Akka Streams are used to handle these dynamic data streams. They allow developers to build robust IoT solutions.
Chat applications require low latency and high concurrency. Messages should appear instantly for both sender and receiver. Reactive programming enables such real-time communication.
Reactive libraries handle the continuous flow of messages effortlessly. This approach ensures that users see messages instantly. Frameworks like Vert.x or Node.js with RxJS are often used to build chat applications.
Financial systems deal with real-time data, such as stock prices and currency rates. Reactive programming helps monitor and react to changes in real time.
For example, a trading platform can react to changes in stock prices. It can automatically execute trades based on predefined rules. Reactive frameworks ensure that these systems operate with minimal latency.
Video streaming platforms like Netflix rely on reactive programming. They use it to manage data streams, handle buffering, and manage user sessions.
Netflix’s API gateway, built on RxJava, enables it to serve millions of users simultaneously. Reactive programming ensures that the platform remains responsive, even under heavy load.
Reactive programming differs significantly from traditional approaches. Here's a comparison:
Blocking vs. Non-Blocking: Traditional programming often involves blocking calls. This approach can lead to inefficiencies. Reactive programming, on the other hand, avoids blocking.
Synchronous vs. Asynchronous: Traditional systems usually follow a synchronous model. Reactive programming embraces asynchronicity, making it better for handling real-time data.
Imperative vs. Declarative: Traditional programming is imperative. It focuses on how to achieve a task. Reactive programming is declarative. It focuses on what to achieve.
These differences make reactive programming more suitable for modern, high-performance applications.
While reactive programming has many benefits, it also has challenges:
Steep Learning Curve: Understanding the concepts can be tough for beginners.
Debugging Complexity: Debugging asynchronous data flows is harder than synchronous ones.
Overhead: Managing multiple data streams can add complexity to the code.
Error Handling: Proper error handling in a reactive system requires careful planning.
Despite these challenges, the benefits often outweigh the drawbacks.
Several libraries and frameworks support reactive programming. Some popular ones include:
RxJava: A reactive library for Java that offers powerful operators and easy integration.
Project Reactor: Built by Pivotal, it’s the core dependency for Spring WebFlux.
Akka Streams: A powerful toolkit for building reactive applications in Scala.
RxJS: A reactive library for JavaScript, used extensively in Angular applications.
These tools make building reactive applications easier and more efficient.
Reactive programming offers a new way to handle data and events. It’s about embracing asynchronicity, responsiveness, and scalability. By leveraging libraries like RxJava or frameworks like Spring WebFlux, developers can build systems that meet modern requirements.
Its use cases span various domains, from UI development to real-time data processing. While it has a steep learning curve, the benefits of reactive programming make it a valuable skill for developers. In a world where real-time interaction is crucial, reactive programming stands out as a powerful approach.