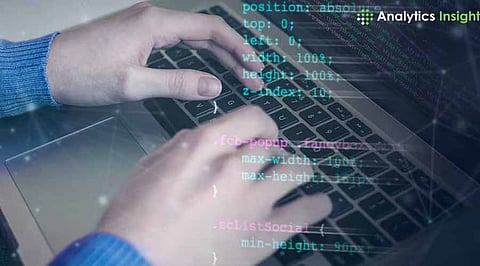
The Document Object Model (DOM) is a programming interface that represents the structure of a document (usually HTML or XML) as a tree of objects. Each element in the document is represented as a node in this tree, which can be manipulated using JavaScript.
A document object model represents the document that is currently loaded in each tab of your browser. This "tree structure" representation is what the browser creates to make the HTML structure easily accessible to programming languages. For instance, the browser uses it to apply styling and other data to the appropriate elements during page rendering, and developers can use JavaScript to manipulate the Document Object Model (DOM) after the page has been rendered.
To get started with DOM manipulation, let's look at a real-world example.
a. Make a local duplicate of the accompanying image and the dom-example.html page.
b. Place an element with a “<script></script>” immediately above the last tag.
c. An element must first be selected and a reference to it must be kept in a variable before it can be altered within the DOM. Put this line within your script element:
jsCopy to Clipboard
const link = document.querySelector("a");
d. Using the properties and methods that are available to the element reference now that it is stored in a variable, we can begin modifying it. These are defined on interfaces such as HTMLAnchorElement, which pertains to elements, HTMLElement, its more general parent interface, and Node, which represents all nodes in a DOM. Let's start by altering the text within the link by changing the Node.textContent property's value. Under the preceding line, insert the following one:
jsCopy to Clipboard
link.textContent = "Mozilla Developer Network";
e. To prevent the link from leading to the incorrect location when clicked, we need to update the URL that the link points to. Put one more line at the bottom, as follows:
jsCopy to Clipboard
link.href = "https://developer.mozilla.org";
As with many things in JavaScript, take note that there are numerous methods for choosing an element and assigning a reference to it to a variable. The current best practice is Document.querySelector(). It lets you use CSS selectors to choose elements, it is convenient. The first element in the document will match the querySelector() call that was made above. Document.querySelectorAll() finds every element in the document that matches the selector and stores references to them in an object that resembles an array called a NodeList. This function can be used to match and manipulate numerous elements.
Any JavaScript developer who wants to construct dynamic and interactive web apps has to grasp the Document Object Model (DOM). You may successfully alter the elements of your HTML document by using the structured representation that the Document Object Model (DOM) offers. Using hands-on exercises, such as changing link text and properties, you may directly witness the power and flexibility of the DOM.
It is simpler to design responsive user interfaces when you can pick and alter items in your document with efficiency by using methods like document.querySelector() and document.querySelectorAll(). Comprehending these ideas not only improves your proficiency with web pages but also establishes the groundwork for more complex web development subjects.