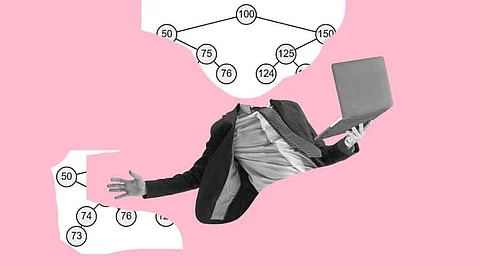
As a Java programmer, understanding data structures is crucial for efficient software development. Data structures are essential for organizing and managing data in your applications. They can significantly impact the performance and functionality of your code. In this article, we'll explore five fundamental data structures that Java programmers need to know.
Arrays are one of the simplest and most commonly used data structures in Java. They are a collection of elements of a similar data type that are stored in contiguous memory locations. Arrays have a fixed size, making them suitable for scenarios where the number of features doesn't change during the program's execution. Accessing elements in an array is done in constant time (O(1)), making it an efficient choice for random access.
A data structure that adheres to the Last-In-First-Out (LIFO) concept is a stack. Java's Stack class or Deque interface may be used to implement stacks. Stacks are commonly used for managing function calls, undo operations, and parsing expressions.
Queues, in contrast to stacks, follow the First-In-First-Out (FIFO) principle. Java offers several implementations of queues, including LinkedList and PriorityQueue. Queues are essential for tasks like managing tasks in a job queue, scheduling processes, and implementing breadth-first search algorithms.
Maps are key-value data structures that allow you to store and retrieve values associated with unique keys. Two widely used map implementations in Java are HashMap and TreeMap. HashMap provides fast access to values by their keys, while TreeMap maintains keys in sorted order.
Lists are dynamic data structures in Java that allow you to store a collection of elements. Two common implementations of lists are ArrayList and LinkedList. ArrayList provides fast access to elements using an index similar to arrays. LinkedList, on the other hand, uses nodes connected by pointers, allowing for efficient insertions and deletions at any position within the list.
Join our WhatsApp Channel to get the latest news, exclusives and videos on WhatsApp
_____________
Disclaimer: Analytics Insight does not provide financial advice or guidance. Also note that the cryptocurrencies mentioned/listed on the website could potentially be scams, i.e. designed to induce you to invest financial resources that may be lost forever and not be recoverable once investments are made. You are responsible for conducting your own research (DYOR) before making any investments. Read more here.